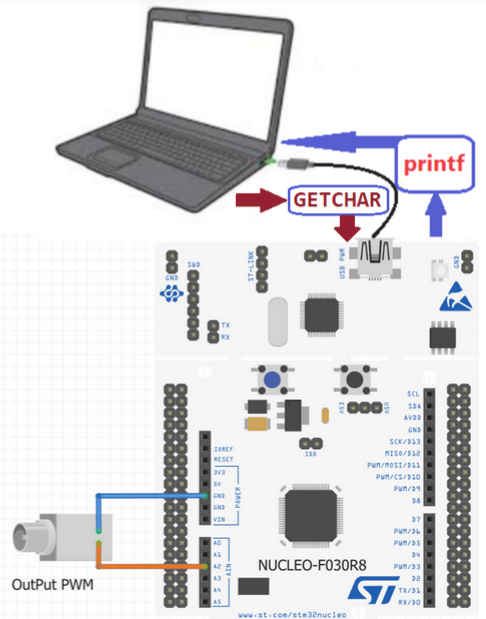
Explanations
This example is based on NUCLEO-F030R8 and STM32CubeIDE and show how to:
- Redirect the printf to USART2
- Getchar via USART2 in Interrupt mode
- Timer (TIM14) in PWM mode at 50% of duty cycle
Timer in PWM mode at 50% of Duty Cycle
For this example we use the TIM14 in PWM output mode with 50% of Duty Cycle.
First is necessary configure the TIM14 but is also necessary enable it in your main.c file.
Insert from:
/* USER CODE BEGIN WHILE */
and
/* USER CODE END WHILE */
the line below:
// Enable Timer (TIM14) for generate PWM (on PA4)
HAL_TIM_PWM_Start(&htim14, TIM_CHANNEL_1);
Below there is an example of IOC configuration for obtain a PWM of 100Hz with Duty of 50%
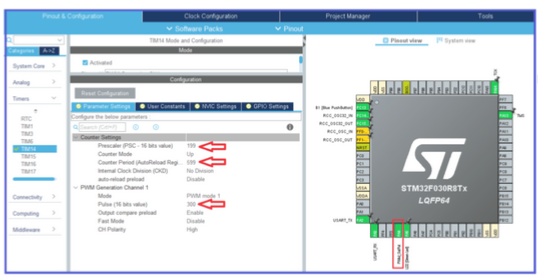
The parameter before are calculated thanks to this spreadsheet (is in LibreOffice format, so if you don’t have install it, it’s free).
For use the spreadsheet you must insert your data in the yellow box, see below.
It’s important choose a PRESCALER value in relation at the Frequency that you need to generate. In other words, choose PRESCALER for obtain a congruent values (integer value).
For this reason we use the STM32F0R8 at 48 Mhz, bat we set the APB1 Timer clocks at 12 Mhz, see below the clock configuration image.

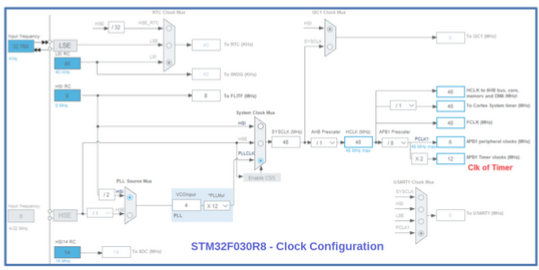
Redirect printf
For redirect output of the printf via UART2 first is necessary configure USART2 next is necessary redirect putchar.
In main.c insert the code below.
Insert from:
/* USER CODE BEGIN Includes */
and
/* USER CODE END Includes */
the line below
#include <stdio.h>
Insert from:
/* USER CODE BEGIN 4 */
and
/* USER CODE END 4 */
the lines below
// Redirect printf on USART2
int __io_putchar(int ch)
{
uint8_t c[1];
c[0] = ch & 0x00FF;
HAL_UART_Transmit(&huart2, &*c, 1, 10);
return ch;
}
int _write(int file,char ptr, int len)
{
int DataIdx;
for(DataIdx= 0; DataIdx< len; DataIdx++)
{
__io_putchar(ptr++);
}
return len;
}
Getchar via UART2 in Interrupt mode
For Getchar via UART2 first is necessary configure USART2 but is also necessary enable it in your main.c
Last but not least, is necessary write a callback for capture the character received from the USART2.
In main.c insert the code below.
Insert from:
/* USER CODE BEGIN WHILE */
and
/* USER CODE END WHILE */
the line below:
// Enable the USART2 in RX mode under Interrupt
HAL_UART_Receive_IT(&huart2, CharDataBuffer, 1);
Insert from:
/* USER CODE BEGIN 4 */
and
/* USER CODE END 4 */
the lines below:
// RX char from USART2 in Interrupt mode
void HAL_UART_RxCpltCallback(UART_HandleTypeDef *huart)
{
RxData=TRUE;
// Restart USART in Rx in Interrupt mode
HAL_UART_Receive_IT(&huart2, CharDataBuffer, 1);
}
NOTE:
We use the RxData in while (1) loop, to handle the characters received, see example below.
// Show the last character received via USART2
if (RxData==TRUE)
{
printf(" ... Last character received (via USART2) is: -> %c <- \n\r", CharDataBuffer[0]);
RxData=FALSE;
}
How to get the SW for this project
Get the SW clicking here.
Power Supply NOTE:
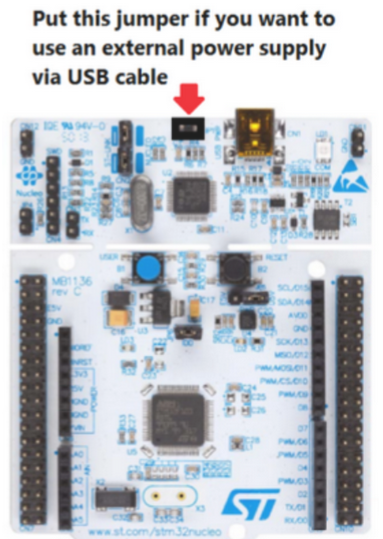
Link